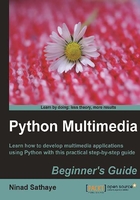
上QQ阅读APP看书,第一时间看更新
Time for action – rotating
- Download the file
Rotate.png
from the Packt website. Alternatively, you can use any supported image file of your choice. - Write the following code in Python interpreter or in a Python file. As always, specify the appropriate path strings for
inPath
andoutPath
variables.1 import Image 2 inPath = "C:\\images\\Rotate.png" 3 img = Image.open(inPath) 4 deg = 45 5 filterOpt = Image.BICUBIC 6 outPath = "C:\\images\\Rotate_out.png" 7 foo = img.rotate(deg, filterOpt) 8 foo.save(outPath)
- Upon running this code, the output image, rotated by 45 degrees, is saved to the
outPath
. The filter optionImage.BICUBIC
ensures highest quality. The next illustration shows the original and the images rotated by 45 and 180 degrees respectively—the original and rotated images. - There is another way to accomplish rotation for certain angles by using the
Image.transpose
functionality. The following code achieves a 270-degree rotation. Other valid options for rotation areImage.ROTATE_90
andImage.ROTATE_180
.import Image inPath = "C:\\images\\Rotate.png" img = Image.open(inPath) outPath = "C:\\images\\Rotate_out.png" foo = img.transpose(Image.ROTATE_270) foo.save(outPath)
What just happened?
In the previous section, we used Image.rotate
to accomplish rotating an image by the desired angle. The image filter Image.BICUBIC
was used to obtain better quality output image after rotation. We also saw how Image.transpose
can be used for rotating the image by certain angles.
Flipping
There are multiple ways in PIL to flip an image horizontally or vertically. One way to achieve this is using the Image.transpose
method. Another option is to use the functionality from the ImageOps
module . This module makes the image-processing job even easier with some ready-made methods. However, note that the PIL documentation for Version 1.1.6 states that ImageOps
is still an experimental module.