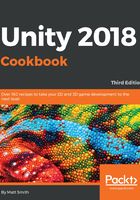
上QQ阅读APP看书,第一时间看更新
How to do it...
To change the pitch of an audio clip according to the speed of an animated object, please follow these steps:
- Create a new Unity 3D project.
- Create a new Models folder in the Project panel, and into this import the provided animatedRocket.fbx file.
- Create a new Sounds folder in the Project panel, and into this Import the provided audio clip, engineSound.wav.
- Select the animatedRocket file in the Project panel. In the Inspector for animatedRocket Import Settings, click the Animations button. In Animations select (the only) Take 001 clip, and make sure to check the Loop Time option. Click on the Apply button to save the changes. See the screenshot for these settings:

The reason we didn't need to check Loop Pose option is because our animation already loops in a seamless fashion. If it didn't, we could have checked that option to automatically create a seamless transition from the last to the first frame of the animation.
- Add an instance of animatedRocket as a GameObject in the scene by dragging it from the Project panel into the Scene or Hierarchy panel.
- Add an AudioSource component to the engineSound GameObject.
- With engineSound selected in the Hierarchy, drag the engineSound AudioClip file from the Project panel into the Inspector for the Audio Clip parameter of the Audio Source component. Ensure the Loop option is checked, and the Play On Awake option is unchecked:

- Create an Animator Controller for our model. Select the Models folder in the Project panel, and use the Create menu to create a new Animator Controller file named Rocket Controller.
- Double-click the Rocket Controller file in the Project panel to open the Animator panel. Create a new state by choosing menu option: Create State | Empty (as shown in the screenshot):

- Rename this new state spin (in its Inspector properties), and set Take 001 as its motion in the Motion field:

- Select animatedRocket in the Hierarchy panel. Drag Rocket Controller from the Models folder in the Project panel into the Controller parameter for the Animator component in the Inspector. Ensure that the Apply Root Motion option is unchecked in the Inspector:

- Create a C# script class, ChangePitch, in the _Scripts folder, containing the following code, and add an instance as a scripted component to the animatedRocket GameObject:
using UnityEngine; public class ChangePitch : MonoBehaviour{ public float acceleration = 0.05f; public float minSpeed = 0.0f; public float maxSpeed = 2.0f; public float animationSoundRatio = 1.0f; private float speed = 0.0f; private Animator animator; private AudioSource audioSource; private void Awake() { animator = GetComponent<Animator>(); audioSource = GetComponent<AudioSource>(); } void Start() { speed = animator.speed; AccelerateRocket (0); } void Update() { if (Input.GetKey (KeyCode.Alpha1)) AccelerateRocket(acceleration); if (Input.GetKey (KeyCode.Alpha2)) AccelerateRocket(-acceleration); } public void AccelerateRocket(float acceleration) { speed += acceleration; speed = Mathf.Clamp(speed,minSpeed,maxSpeed); animator.speed = speed; float soundPitch = animator.speed * animationSoundRatio; audioSource.pitch = Mathf.Abs(soundPitch); } }
- Play the scene and change the animation speed by pressing number key 1 (accelerate) and 2 (decelerate) on your keyboard. The audio pitch will change accordingly.