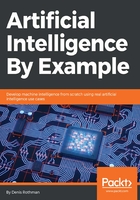
Designing the architecture of the data flow graph
First import TensorFlow with the following code:
import tensorflow as tf
Then use the following code to give a name of the scope of each object you want to display in one line. Write your variable or object on the second line and include it in the TensorBoard summary as an image (histograms, scalars, and other summaries are possible), as shown in the following code:
with tf.name_scope("Input"):
x_ = tf.placeholder(tf.float32, shape=[4,2], name = 'x-input-predicates')
tf.summary.image('input predicates x', x_, 10)
name provides a label for the objects in the graph. scope is a way to group the names to simplify the visual representation of your architecture.
Repeat that with all the elements described in the previous section, as follows.
with tf.name_scope("Input_expected_output"):
y_ = tf.placeholder(tf.float32, shape=[4,1], name = 'y-expected-output')
tf.summary.image('input expected values of y', x_, 10)
...
with tf.name_scope("Error_Loss"):
cost = tf.reduce_mean(( (y_ * tf.log(Output)) + ((1 - y_) * tf.log(1.0 - Output)) ) * -1)
tf.summary.image("Error_Loss", cost, 10)
Then, once the session is created, set up the log writer directory, as shown in the following code:
init = tf.global_variables_initializer()
sess = tf.Session()
writer = tf.summary.FileWriter("./logs/tensorboard_logs", sess.graph)
sess.run(init)
#THE PROGRAM DATE FEED CODE GOES HERE. See FNN_XOR_Tensorflow.py
writer.close()
Those are the basic steps to create the logs to display in TensorBoard.
Note that this source code only contains the architecture of the data flow graph and no program running the data. The program is the architecture that defines computations. The rest is defining the datasets—how they will be fed (totally or partially) to the graph. I recommend that you use TensorBoard to design the architecture of your solutions with the TensorFlow graph code. Only then, introduce the dynamics of feeding data and running a program.