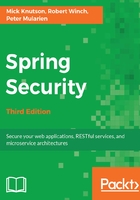
Implementing a Spring Security XML configuration file
The next step in the configuration process is to create a Java configuration file representing all Spring Security components required to cover standard web requests.
Create a new Java file in the src/main/java/com/packtpub/springsecurity/configuration/ directory with the name SecurityConfig.java, and the following content. Among other things, the following file demonstrates user login requirements for every page in our application, provides a login page, authenticates the user, and requires the logged-in user to be associated with a role called USER for every URL element:
//src/main/java/com/packtpub/springsecurity/configuration/
SecurityConfig.java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
public void configure(final AuthenticationManagerBuilder auth) throws Exception
{
auth.inMemoryAuthentication().withUser("user1@example.com")
.password("user1").roles("USER");
}
@Override
protected void configure(final HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/**").access("hasRole('USER')")
// equivalent to <http auto-config="true">
.and().formLogin()
.and().httpBasic()
.and().logout()
// CSRF is enabled by default (will discuss later)
.and().csrf().disable();
}
}
If you are using Spring Tool Suite, you can easily review WebSecurityConfigurerAdapter by using F3. Remember that the next checkpoint (chapter02.01-calendar) has a working solution, so the file can be copied from there as well.
This is the only Spring Security configuration required to get our web application secured with a minimal standard configuration. This style of configuration, using a Spring Security-specific Java configuration, is known as Java Config.
Let's take a minute to break this configuration apart so we can get a high-level idea of what is happening. In the configure(HttpSecurity) method, the HttpSecurity object creates a Servlet Filter, which ensures that the currently logged-in user is associated with the appropriate role. In this instance, the filter will ensure that the user is associated with ROLE_USER. It is important to understand that the name of the role is arbitrary. Later, we will create a user with ROLE_ADMIN and will allow this user to have access to additional URLs that our current user does not have access to.
In the configure(AuthenticationManagerBuilder) method, the AuthenticationManagerBuilder object is how Spring Security authenticates the user. In this instance, we utilize an in-memory data store to compare a username and password.
Our example and explanation of what is happening are a bit contrived. An in-memory authentication store would not work in a production environment. However, it allows us to get things up and running quickly. We will incrementally improve our understanding of Spring Security as we update our application to use production quality security throughout this book.
General support for Java Configuration was added to Spring Framework in Spring 3.1. Since Spring Security 3.2 release, there has been Spring Security Java Configuration support, which enables users to easily configure Spring Security without the use of any XML. If you are familiar with Chapter 6, LDAP Directory Services, and the Spring Security documentation, then you should find quite a few similarities between it and Security Java Configuration support.