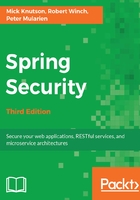
Conditionally displaying authentication information
Currently, our application has no indication as to whether we are logged in or not. In fact, it appears as though we are always logged in since the Logout link is always displayed. In this section, we will demonstrate how to display the authenticated user's username and conditionally display portions of the page using Thymeleaf’s Spring Security tag library. We do so by performing the following steps:
- Update your dependencies to include the thymeleaf-extras-springsecurity4 JAR file. Since we are using Gradle, we will add a new dependency declaration in our build.gradle file, as follows:
//build.gradle
dependency{
...
compile 'org.thymeleaf.extras:thymeleaf-
extras-springsecurity4'
}
- Next, we need to add SpringSecurityDialect to the Thymeleaf engine as follows:
//src/com/packtpub/springsecurity/web/configuration/
ThymeleafConfig.java
@Bean
public SpringTemplateEngine templateEngine(
final ServletContextTemplateResolver resolver)
{
SpringTemplateEngine engine = new SpringTemplateEngine();
engine.setTemplateResolver(resolver);
engine.setAdditionalDialects(new HashSet<IDialect>() {{
add(new LayoutDialect());
add(new SpringSecurityDialect());
}});
return engine;
}
- Update the header.html file to leverage the Spring Security tag library. You can find the updates as follows:
//src/main/webapp/WEB-INF/templates/fragments/header.html
<html xmlns:th="http://www.thymeleaf.org"
xmlns:sec="http://www.thymeleaf.org/thymeleaf-
extras-springsecurity4">
...
<p id="navbar" class="collapse navbar-collapse">
...
<ul class="nav navbar-nav pull-right"
sec:authorize="isAuthenticated()">
<li>
<p class="navbar-text">Welcome <p class="navbar-text"
th:text="${#authentication.name}">User</p></p>
</li>
<li>
<a id="navLogoutLink" class="btn btn-default"
role="button" th:href="@{/logout}">Logout</a>
</li>
<li> | </li>
</ul>
<ul class="nav navbar-nav pull-right"
sec:authorize=" ! isAuthenticated()">
<li><a id="navLoginLink" class="btn btn-default"
role="button"
th:href="@{/login/form}">Login</a></li>
<li> | </li>
</ul>
...
The sec:authorize attribute determines whether the user is authenticated with the isAuthenticated()value, and displays the HTML node if the user is authenticated, and hides the node in the event that the user is not authenticated. The access attribute should be rather familiar from the antMatcher().access() element. In fact, both components leverage the same SpEL support. There are attributes in the Thymeleaf tag libraries that do not use expressions. However, using SpEL is typically the preferred method since it is more powerful.
The sec:authentication attribute will look up the current o.s.s.core.Authentication object. The property attribute will find the principal attribute of the o.s.s.core.Authentication,object, which in this case is o.s.s.core.userdetails.UserDetails. It then obtains the UserDetails username property and renders it to the page. Don't worry if the details of this are confusing. We are going to go over this in more detail in Chapter 3, Custom Authentication.
If you haven't done so already, restart the application to see the updates we have made. At this point, you may realize that we are still displaying links we do not have access to. For example, user1@example.com should not see a link to the All Events page. Rest assured, we'll fix this when we cover the tags in greater detail in Chapter 11, Fine-Grained Access Control.
Your code should now look like this: chapter02.05-calendar.