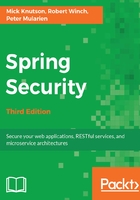
The SpringSecurityUserContext simplifications
We have updated CalendarUserDetailsService to return a UserDetails object that extends CalendarUser and implements UserDetails. This means that, rather than having to translate between the two objects, we can simply refer to a CalendarUser object. Update SpringSecurityUserContext as follows:
public class SpringSecurityUserContext implements UserContext {
public CalendarUser getCurrentUser() {
SecurityContext context = SecurityContextHolder.getContext();
Authentication authentication = context.getAuthentication();
if(authentication == null) {
return null;
}
return (CalendarUser) authentication.getPrincipal();
}
public void setCurrentUser(CalendarUser user) {
Collection authorities =
CalendarUserAuthorityUtils.createAuthorities(user);
Authentication authentication = new UsernamePasswordAuthenticationToken(user,user.getPassword(), authorities);
SecurityContextHolder.getContext()
.setAuthentication(authentication);
}
}
The updates no longer require the use of CalendarUserDao or Spring Security's UserDetailsService interface. Remember our loadUserByUsername method from the previous section? The result of this method call becomes the principal of the authentication. Since our updated loadUserByUsername method returns an object that extends CalendarUser, we can safely cast the principal of the Authentication object to CalendarUser. We can pass a CalendarUser object as the principal into the constructor for UsernamePasswordAuthenticationToken when invoking the setCurrentUser method. This allows us to still cast the principal to a CalendarUser object when invoking the getCurrentUser method.