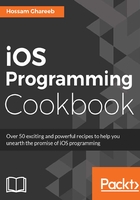
Delegation
Delegation is the most commonly used design pattern in iOS. In delegation, you enable types to delegate some of its responsibilities or functions to another instance of another type. To create this design pattern, we use protocols that will contain the list of responsibilities or functions to be delegated. We usually use delegation when you want to respond to actions or retrieve or get information from other sources without needing to know the type of that sources, except that they conform to that protocol. Let's take a look at an example of how to create use delegate:
@objc protocol DownloadManagerDelegate { func didDownloadFile(fileURL: String, fileData: NSData) func didFailToDownloadFile(fileURL: String, error: NSError) } class DownloadManager{ weak var delegate: DownloadManagerDelegate! func downloadFileAtURL(url: String){ // send request to download file // check response and success or failure if let delegate = self.delegate { delegate.didDownloadFile(url, fileData: NSData()) } } } class ViewController: UIViewController, DownloadManagerDelegate{ func startDownload(){ letdownloadManager = DownloadManager() downloadManager.delegate = self } func didDownloadFile(fileURL: String, fileData: NSData) { // present file here } func didFailToDownloadFile(fileURL: String, error: NSError) { // Show error message } }
The protocol DownloadManagerDelegate contains methods that would be called once the specific actions happen to inform the class that conforms to that protocol. The DownloadManager class performs the download tasks asynchronously and informs the delegate with success or failure after it's completed. DownloadManager doesn't need to know which object will use it or any information about it. The only thing it cares about is that the class should conform to the delegate protocol, and that's it.